Properties file (.properties) chủ yếu được sử dụng trong các công nghệ liên quan đến Java để lưu trữ các tham số có thể cấu hình của một ứng dụng. Properties là các giá trị được quản lý theo các cặp key/value. Trong mỗi cặp, key và value đều có kiểu String. Key được sử dụng để truy xuất value, giống như tên biến được sử dụng để truy xuất giá trị của biến.
Bài này hướng dẫn bạn cách read properties file trong java:
- Read properties file từ thư mục hiện tại.
- Read properties file từ resources (classpath).
- Read properties file bằng cách sử dụng Singleton pattern.
Nội dung chính
Định nghĩa file properties
File properties có nội dung như sau, được sử dụng trong các ví dụ của bài này:
username=admin password=123456
1. Read properties file từ thư mục hiện tại
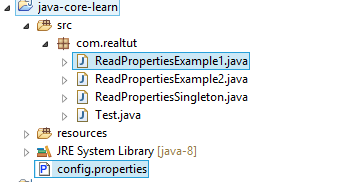
Tạo file config.properties trong cùng thư mục với java resources.
File: ReadPropertiesExample1.java
package com.realtut; import java.io.FileInputStream; import java.io.IOException; import java.io.InputStream; import java.util.Properties; public class ReadPropertiesExample1 { private static final String FILE_CONFIG = "\\config.properties"; public static void main(String[] args) { Properties properties = new Properties(); InputStream inputStream = null; try { String currentDir = System.getProperty("user.dir"); inputStream = new FileInputStream(currentDir + FILE_CONFIG); // load properties from file properties.load(inputStream); // get property by name System.out.println(properties.getProperty("username")); System.out.println(properties.getProperty("password")); } catch (IOException e) { e.printStackTrace(); } finally { // close objects try { if (inputStream != null) { inputStream.close(); } } catch (IOException e) { e.printStackTrace(); } } } }
Kết quả:
admin 123456
2. Read properties file từ resources (classpath)
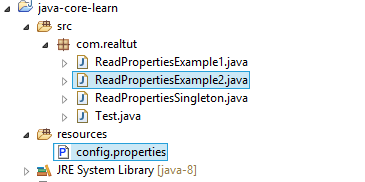
Tạo file config.properties trong java resources.
File: ReadPropertiesExample2.java
package com.realtut; import java.io.IOException; import java.io.InputStream; import java.util.Properties; public class ReadPropertiesExample2 { private static final String FILE_CONFIG = "\\config.properties"; public static void main(String[] args) { Properties properties = new Properties(); InputStream inputStream = null; try { inputStream = ReadPropertiesExample2.class.getClassLoader() .getResourceAsStream(FILE_CONFIG); // load properties from file properties.load(inputStream); // get property by name System.out.println(properties.getProperty("username")); System.out.println(properties.getProperty("password")); } catch (IOException e) { e.printStackTrace(); } finally { // close objects try { if (inputStream != null) { inputStream.close(); } } catch (IOException e) { e.printStackTrace(); } } } }
Kết quả:
admin 123456
3. Read properties file sử dụng Singleton pattern
File: ReadPropertiesSingleton.java
package com.realtut; import java.io.FileInputStream; import java.io.IOException; import java.io.InputStream; import java.util.Properties; public class ReadPropertiesSingleton { private static final String FILE_CONFIG = "\\config.properties"; private static ReadPropertiesSingleton instance = null; private Properties properties = new Properties(); /** * Use singleton pattern to create ReadConfig object one time and use * anywhere * * @return instance of ReadConfig object */ public static ReadPropertiesSingleton getInstance() { if (instance == null) { instance = new ReadPropertiesSingleton(); instance.readConfig(); } return instance; } /** * get property with key * * @param key * @return value of key */ public String getProperty(String key) { return properties.getProperty(key); } /** * read file .properties */ private void readConfig() { InputStream inputStream = null; try { String currentDir = System.getProperty("user.dir"); inputStream = new FileInputStream(currentDir + FILE_CONFIG); properties.load(inputStream); } catch (IOException e) { e.printStackTrace(); } finally { // close objects try { if (inputStream != null) { inputStream.close(); } } catch (IOException e) { e.printStackTrace(); } } } }
File: Test.java
package com.realtut; public class Test { public static void main(String[] args) { ReadPropertiesSingleton demo = ReadPropertiesSingleton.getInstance(); System.out.println(demo.getProperty("username")); System.out.println(demo.getProperty("password")); } }
Kết quả:
admin 123456