Component trong Angular về cơ bản là các lớp tương tác với file .html, được hiển thị trên trình duyệt. Bài này chúng ta sẽ bàn luận về cách tạo và sử dụng component trong Angular.
Nội dung chính
Cấu trúc tập tin của một Component
Cấu trúc tập tin của app component trong bài học trước như sau:
- app.component.css
- app.component.html
- app.component.spec.ts
- app.component.ts
- app.module.ts
Và nếu bạn đã chọn angular routing trong quá trình tạo dự án, các file liên quan đến routing cũng sẽ được thêm vào như sau:
- app-routing.module.ts
Các file trên được tạo theo mặc định khi chúng ta tạo dự án mới bằng lệnh angular-cli.
Giới thiệu file app.module.ts
File app.module.ts có nội dung như sau:
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppRoutingModule } from './app-routing.module'; import { AppComponent } from './app.component'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, AppRoutingModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
Trong đó:
- @NgModule: để định nghĩa Class AppModule là một Angular Module.
- @NgModule: cũng sẽ có những metadata object, cái mà sẽ nói cho Angular biết cách biên dịch và khởi chạy ứng dụng của chúng ta.
- Imports: import BrowserModule module, module này cần cho mọi ứng dụng chạy trên trình duyệt.
- Declarations: Khai báo những component sẽ được sử dụng trong module này.
- Boostrap: Component gốc được Angular tạo ra và chèn vào trong index.html.
Tạo component trong Angular
Chúng ta sẽ sử dụng lệnh angular-cli để tạo component mới. Tuy nhiên, app component được tạo theo mặc định sẽ luôn là component cha và các component tiếp theo được tạo sẽ tạo thành các component con.
ng g component new-cmp
Khi bạn chạy lệnh trên trong command line, bạn sẽ nhận được kết quả sau:
C:\Angular\Angular7\my-app>ng g component new-cmp CREATE src/app/new-cmp/new-cmp.component.html (26 bytes) CREATE src/app/new-cmp/new-cmp.component.spec.ts (629 bytes) CREATE src/app/new-cmp/new-cmp.component.ts (272 bytes) CREATE src/app/new-cmp/new-cmp.component.css (0 bytes) UPDATE src/app/app.module.ts (477 bytes)
Bây giờ, chúng ta kiểm tra cấu trúc file, chúng ta sẽ có một thư mục mới new-cmp được tạo trong thư mục src/app. Có các file như sau:
- new-cmp.component.css − tệp css cho component mới được tạo.
- new-cmp.component.html − tệp html được tạo.
- new-cmp.component.spec.ts − cái này có thể được sử dụng cho unit testing.
- new-cmp.component.ts − ở đây, chúng ta có thể định nghĩa mô-đun, thuộc tính, v.v.
File app.module.ts được cập nhật như sau:
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppRoutingModule } from './app-routing.module'; import { AppComponent } from './app.component'; import { NewCmpComponent } from './new-cmp/new-cmp.component'; @NgModule({ declarations: [ AppComponent, NewCmpComponent ], imports: [ BrowserModule, AppRoutingModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
File new-cmp.component.ts được tạo như sau:
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-new-cmp', templateUrl: './new-cmp.component.html', styleUrls: ['./new-cmp.component.css'] }) export class NewCmpComponent implements OnInit { constructor() { } ngOnInit() { } }
Bạn để ý thấy file new-cmp.component.ts ở trên, nó sẽ tạo một lớp mới gọi là NewCmpComponent, triển khai lớp OnInit, trong đó có một hàm constructor() và một phương thức ngOnInit(). Phương thức ngOnInit() sẽ được gọi theo mặc định khi lớp được thực thi.
Luồng thực thi của ứng dụng Angular
Tiếp theo chúng ta sẽ kiểm tra luồng thực thi của ứng dụng Angular hoạt động như thế nào. Bây giờ, app component, được tạo theo mặc định sẽ trở thành component chính. Các component được thêm vào sau đó sẽ trở thành component con.
Khi chúng ta gõ URL "http://localhost:4200/" trong trình duyệt, trước tiên hệ thống sẽ thực thi file my-app\src\index.html, file này có nội dung như sau:
<!doctype html> <html lang="en"> <head> <meta charset="utf-8"> <title>MyApp</title> <base href="/"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="icon" type="image/x-icon" href="favicon.ico"> </head> <body> <app-root></app-root> </body> </html>
Nó chứa thẻ app-root trong body như sau:
<app-root></app-root>
Đây là thẻ gốc được tạo bởi Angular theo mặc định. Thẻ này có tham chiếu trong tệp main.ts.
import { enableProdMode } from '@angular/core'; import { platformBrowserDynamic } from '@angular/platform-browser-dynamic'; import { AppModule } from './app/app.module'; import { environment } from './environments/environment'; if (environment.production) { enableProdMode(); } platformBrowserDynamic().bootstrapModule(AppModule) .catch(err => console.error(err));
AppModule được import từ app của module chính, và điều tương tự bootstrap module, làm cho app module được tải.
File app.module.ts :
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppRoutingModule } from './app-routing.module'; import { AppComponent } from './app.component'; import { NewCmpComponent } from './new-cmp/new-cmp.component'; @NgModule({ declarations: [ AppComponent, NewCmpComponent ], imports: [ BrowserModule, AppRoutingModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
Ở đây, AppComponent là tên được đặt sau từ khóa export class
, tức là biến để lưu trữ tham chiếu của app.component.ts và tương tự được đặt cho bootstrap. Bây giờ chúng ta hãy xem file app.component.ts.
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 7'; }
Angular core được import và được gọi là Component và tương tự được sử dụng trong Declarator như sau:
@Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] })
selector, templateUrl và styleUrls được gọi là decorators. Trong đó selector là 'app-root' chính là thẻ được đặt trong file index.html mà chúng ta đã thấy ở trên.
Lớp AppComponent có một biến gọi là title, được hiển thị trong trình duyệt. @Component sử dụng templateUrl được gọi là app.component.html như sau:
<!--The content below is only a placeholder and can be replaced.--> <div style="text-align:center"> <h1> Welcome to {{ title }}! </h1> </div>
{{ title }} sẽ được thay thế bằng giá trị được định nghĩa trong file app.component.ts , nó được gọi là data binding mà chúng ta sẽ tìm hiểu trong bài sau.
Chúng ta đã tạo ra một component mới có tên là new-cmp. Thành phần mới này đã được khai báo trong file app.module.ts khi chúng ta thực thi lệnh tạo component mới.
app.module.ts có tham chiếu đến component mới được tạo.
Sử dụng component mới được tạo ra trong thư mục app/new-cmp
File new-cmp.component.ts
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-new-cmp', templateUrl: './new-cmp.component.html', styleUrls: ['./new-cmp.component.css'] }) export class NewCmpComponent implements OnInit { constructor() { } ngOnInit() { } }
Trong đó, declarator gồm có selector được gọi là 'app-new-cmp' và templateUrl và styleUrls.
File new-cmp.component.html có nội dung như sau:
<p> new-cmp works! </p>
File new-cmp.component.css có nội dung trống, bạn có thể update nội dung như sau:
p { color: red; }
Nhưng khi chúng ta chạy dự án, chúng ta sẽ không thấy bất cứ điều gì liên quan đến component mới được hiển thị trong trình duyệt:
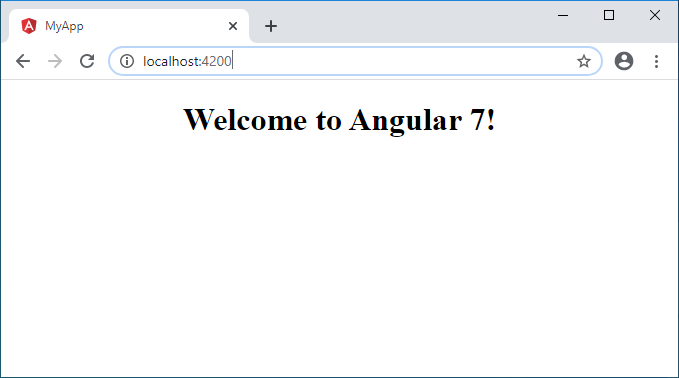
Như bạn đã thấy trong file new-cmp.component.ts có khai báo selector: 'app-new-cmp'
. Selector, tức là chúng ta sẽ tương tác với component này thông qua thẻ app-new-cmp
.
Bây giờ hãy thêm thẻ
<!--The content below is only a placeholder and can be replaced.--> <div style="text-align:center"> <h1> Welcome to {{ title }}! </h1> <app-new-cmp></app-new-cmp> </div>
Kết quả hiển thị trên trình duyệt:
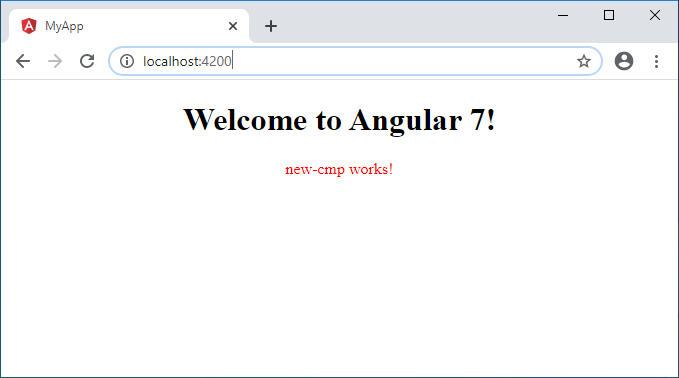
Hãy thêm một số chi tiết cho component mới được tạo bằng cách sửa file new-cmp.component.ts:
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-new-cmp', templateUrl: './new-cmp.component.html', styleUrls: ['./new-cmp.component.css'] }) export class NewCmpComponent implements OnInit { newcomponent = "New Component"; constructor() { } ngOnInit() { } }
Trong class NewCmpComponent, chúng ta thêm một biến gọi là newcomponent và giá trị là "New Component".
Biến trên được thêm vào trong file new-cmp.component.html như sau:
<p> new-cmp works! </p> <p> {{newcomponent}} </p>
Cập nhật file new-cmp.component.css như sau:
p { color: red; font-size: 25px; }
Kết quả hiển thị trên trình duyệt:
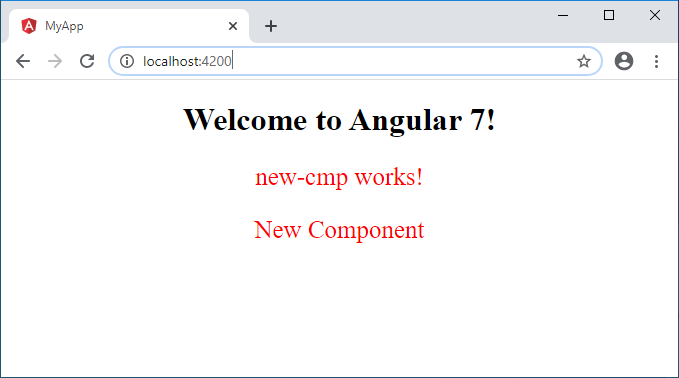
Tương tự, chúng ta có thể tạo các component và liên kết chúng bởi sử dụng selector trong file app.component.html