Nội dung chính
HttpClient trong Angular
HttpClient sẽ giúp chúng ta tìm nạp dữ liệu từ các nguồn bên ngoài, v.v. Chúng ta cần module mô-đun http để sử dụng các service của http.
Ví dụ HttpClient trong Angular
Tiếp tục sử dụng project trong các bài trước.
Để bắt đầu sử dụng http service, chúng ta cần import mô-đun HttpClientModule trong app.module.ts như sau:
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppRoutingModule, RoutingComponent } from './app-routing.module'; import { AppComponent } from './app.component'; import { NewCmpComponent } from './new-cmp/new-cmp.component'; import { AddTextDirective } from './add-text.directive'; import { SqrtPipe, SquarePipe } from './app.sqrt'; import { HomeComponent } from './home/home.component'; import { ContactusComponent } from './contactus/contactus.component'; import { MyserviceService } from './myservice.service'; import { HttpClientModule } from '@angular/common/http'; @NgModule({ declarations: [ AppComponent, NewCmpComponent, AddTextDirective, SqrtPipe, SquarePipe, HomeComponent, ContactusComponent, RoutingComponent ], imports: [ BrowserModule, AppRoutingModule, HttpClientModule ], providers: [MyserviceService], bootstrap: [AppComponent] }) export class AppModule { }
Chúng ta sẽ tìm nạp dữ liệu từ máy chủ bằng mô-đun httpclient. Chúng ta sẽ sử dụng service tạo ở bài trước để thực hiện đọc dữ liệu từ API lấy thông tin user http://jsonplaceholder.typicode.com/users.
myservice.service.ts
import { Injectable } from '@angular/core'; import { HttpClient } from '@angular/common/http'; @Injectable({ providedIn: 'root' }) export class MyserviceService { private finaldata = []; private apiurl = "http://jsonplaceholder.typicode.com/users"; constructor(private httpClient: HttpClient) { } getData() { return this.httpClient.get(this.apiurl); } }
Phương thức getData() trả về dữ liệu được tìm nạp cho url đã cho. Phương thức httpClient.get() trả về một Observable bất đồng bộ.
Phương thức getData được gọi từ app.component.ts như sau:
app.component.ts
import { Component } from '@angular/core'; import { MyserviceService } from './myservice.service'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 7 Project!'; public persondata = []; constructor(private myservice: MyserviceService) {} ngOnInit() { this.myservice.getData().subscribe((data) => { Object.keys(data).forEach(key => { this.persondata.push(data[key]); }); console.log(this.persondata); }); } }
Chúng ta gọi phương thức getData() để trả về một kiểu dữ liệu Observable. Phương thức subscribe() được sử dụng để đăng ký - nghĩa hệ thống sẽ lắng nghe khi nào có dữ liệu trả về Observable tương ứng thì hàm này sẽ được gọi.
Ký hiệu => là biểu thức lambda trong TypeScript. Cú pháp chúng ta sử dụng trong lập trình Angular là TypeScript. Sau đó nó được convert thành JavaScript.
Tiếp theo, hiển thị data lên trình duyệt bằng cách cập nhật file view như sau:
app.component.html
<h3>Users Data</h3> <ul> <li *ngFor="let item of persondata; let i = index"> {{item.name}} </li> </ul>
Kết quả:
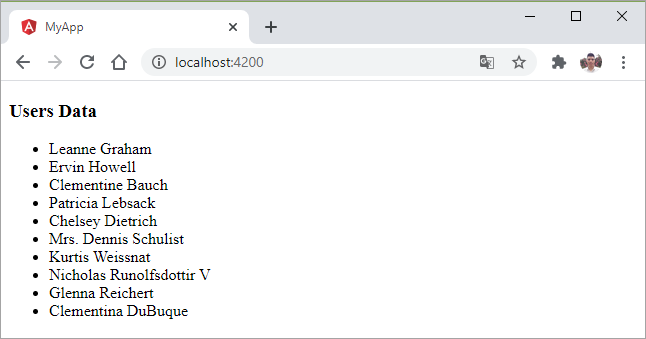
Nhấn phím F12, bạn có thể check console log trong trình duyệt Chrome:
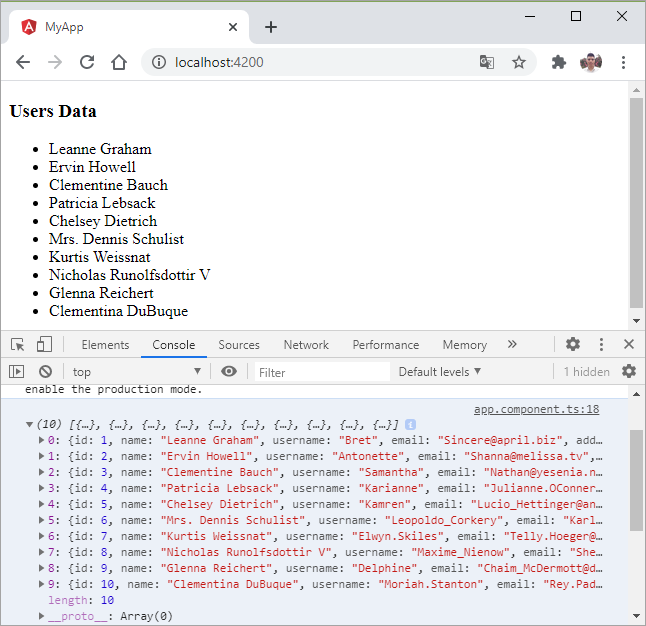